polar.dense#
Classes#
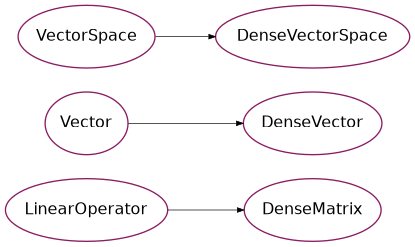
|
|
|
|
|
Space of one-dimensional global arrays, NOT distributed across processes. |
Details#
- class DenseVectorSpace(n, *, dtype=numpy.float64, cart=None, radial_dim=0, angle_dim=1)[source]#
Bases:
VectorSpace
Space of one-dimensional global arrays, NOT distributed across processes.
Typical examples are the right-hand side vector b and solution vector x of a linear system A*x=b.
- Parameters:
- nint
Number of vector components.
- dtypedata-type, optional
Desired data-type for the arrays (default is numpy.float64).
- cartpsydac.ddm.cart.CartDecomposition, optional
N-dimensional Cartesian communicator with N >= 2 (default is None).
- radial_dimint, optional
Dimension index for radial variable (default is 0).
- angle_dimint, optional
Dimension index for angle variable (default is 1).
Notes
The current implementation is tailored to the algorithm for imposing C^1 continuity of a field on a domain with a polar singularity (O-point).
Given an N-dimensional Cartesian communicator (N=2+M), each process belongs to 3 different subcommunicators:
An ‘angular’ 1D subcommunicator, where all processes share the same identical (M+1)-dimensional array;
A ‘radial’ 1D subcommunicator, where only the ‘master’ process has access to the data array (other processes store a 0-length array);
A ‘tensor’ M-dimensional subcommunicator, where the data array is distributed among processes and requires the usual StencilVector communication pattern.
When computing the dot product between two vectors, the following operations will be performed in sequence:
Processes with radial coordinate = 0 compute a local dot product;
Processes with radial coordinate = 0 perform an MPI_ALLREDUCE operation on the ‘tensor’ subcommunicator;
All processes perform an MPI_BCAST operation on the ‘radial’ subcommunicator (process with radial coordinate = 0 is the root);
- property dimension#
The dimension of a vector space V is the cardinality (i.e. the number of vectors) of a basis of V over its base field.
- property dtype#
The data type of the field over which the space is built.
- zeros()[source]#
Get a copy of the null element of the DenseVectorSpace V.
- Returns:
- nullDenseVector
A new vector object with all components equal to zero.
- inner(x, y)[source]#
Evaluate the inner vector product between two vectors of this space V.
If the field of V is real, compute the classical scalar product. If the field of V is complex, compute the classical sesquilinear product with linearity on the second vector.
TODO [YG 01.05.2025]: Currently, the first vector is conjugated. We want to reverse this behavior in order to align with the convention of FEniCS.
TODO [MCP 07.05.2025]: Actually, there is currently no conjugation, since numpy.dot is being called.
- Parameters:
- xVector
The first vector in the scalar product. In the case of a complex field, the inner product is antilinear w.r.t. this vector (hence this vector is conjugated). NOTE [MCP 07.05.2025]: currently without conjugate, see np.dot
- yVector
The second vector in the scalar product. The inner product is linear w.r.t. this vector.
- Returns:
- float | complex
The scalar product of the two vectors. Note that inner(x, x) is a non-negative real number which is zero if and only if x = 0.
- axpy(a, x, y)[source]#
Increment the vector y with the a-scaled vector x, i.e. y = a * x + y, provided that x and y belong to the same vector space V (self). The scalar value a may be real or complex, depending on the field of V.
- Parameters:
- ascalar
The scaling coefficient needed for the operation.
- xVector
The vector which is not modified by this function.
- yVector
The vector modified by this function (incremented by a * x).
- property parallel#
- property ncoeff#
Local number of coefficients.
- property tensor_comm#
- property angle_comm#
- property radial_comm#
- property radial_root#
- class DenseVector(V, data)[source]#
Bases:
Vector
- property space#
Vector space to which this vector belongs.
- copy(out=None)[source]#
Return an identical copy of this vector.
Subclasses must ensure that x.copy(out=x) returns x and not a new object.
- conjugate(out=None)[source]#
Compute the complex conjugate vector.
Please note that x.conjugate(out=x) modifies x in place and returns x.
If the field is real (i.e. self.dtype in (np.float32, np.float64)) this method is equivalent to copy. If the field is complex (i.e. self.dtype in (np.complex64, np.complex128)) this method returns the complex conjugate of self, element-wise.
The behavior of this function is similar to numpy.conjugate(self, out=None).
- property ghost_regions_in_sync#
- class DenseMatrix(V, W, data)[source]#
Bases:
LinearOperator
- property domain#
The domain of the linear operator - an element of Vectorspace
- property codomain#
The codomain of the linear operator - an element of Vectorspace
- transpose(conjugate=False)[source]#
Transpose the LinearOperator .
If conjugate is True, return the Hermitian transpose.
- property dtype#
The data type of the coefficients of the linear operator, upon convertion to matrix.
- dot(v, out=None)[source]#
Apply the LinearOperator self to the Vector v.
The result is written to the Vector out, if provided.
- Parameters:
- vVector
The vector to which the linear operator (self) is applied. It must belong to the domain of self.
- outVector
The vector in which the result of the operation is stored. It must belong to the codomain of self. If out is None, a new vector is created and returned.
- Returns:
- Vector
The result of the operation. If out is None, a new vector is returned. Otherwise, the result is stored in out and out is returned.