ddm.cart#
Functions#
|
This function Connects the Cartesian grids when they share an interface. |
|
Find correct MPI datatype that corresponds to user-provided datatype. |
Classes#
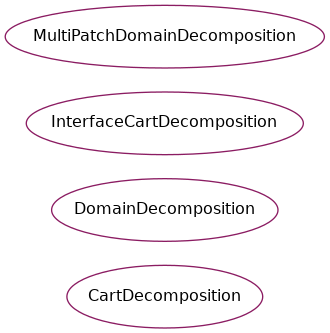
|
Cartesian decomposition of a tensor-product grid of spline coefficients. |
|
Cartesian decomposition of an N-Cube grid. |
|
The Cartesian decomposition of an interface constructed from the Cartesian decomposition of the patches that shares an interface. |
|
Cartesian decomposition of multiple N-Cube grids. |
Details#
- find_mpi_type(dtype)[source]#
Find correct MPI datatype that corresponds to user-provided datatype.
- Parameters:
- dtype[type | str | numpy.dtype | mpi4py.MPI.Datatype]
Datatype for which the corresponding MPI datatype is requested.
- Returns:
- mpi_typempi4py.MPI.Datatype
MPI datatype to be used for communication.
- class MultiPatchDomainDecomposition(ncells, periods, comm=None, num_threads=None)[source]#
Bases:
object
Cartesian decomposition of multiple N-Cube grids. This is built on top of an MPI communicator decomposed into smaller disjoint intra-communicators assigned to each N-Cube grid to construct a multi-dimensional Cartesian topology.
- Parameters:
- ncellslist of list of int
The number of cells in each direction for each grid.
- periods: list of bool
The periodicity of the domain in each direction for each grid.
- commMPI.Comm
MPI communicator that will be used to spawn the grids.
- num_threads: int
Number of threads used by one MPI rank.
- property ncells#
- property periods#
- property size#
- property rank#
- property sizes#
- property rank_ranges#
- property local_groups#
- property local_communicators#
- property owned_groups#
- property domains#
- property num_threads#
- property comm#
- class DomainDecomposition(ncells, periods, comm=None, global_comm=None, num_threads=None, size=None, mpi_dims_mask=None)[source]#
Bases:
object
Cartesian decomposition of an N-Cube grid. This is built on top of an MPI communicator with multi-dimensional Cartesian topology.
- Parameters:
- ncellslist of int
The number of cells in each direction.
- periods: list of bool
The periodcity of the domain in each direction.
- commMPI.Comm|None
MPI communicator that will be used to spawn a new Cartesian communicator. In the serial case comm == None.
- global_commMPI.Comm|None
MPI global communicator that contains all the processes owned by comm. In the serial case comm == None.
- num_threads: int|None
Number of threads used by one MPI rank.
- size: int|None
The number of processes assigned to the domain. This information is needed when comm is None (sequential case) or comm == MPI.COMM_NULL (MPI rank does not own the domain), to be able to calculate global_element_starts and global_element_ends.
- mpi_dims_mask: list of bool
True if the dimension is to be used in the domain decomposition (=default for each dimension). If mpi_dims_mask[i]=False, the i-th dimension will not be decomposed.
- property ndim#
- property ncells#
- property periods#
- property size#
- property rank#
- property num_threads#
- property comm#
- property comm_cart#
- property global_comm#
- property nprocs#
- property global_element_starts#
- property global_element_ends#
- property is_comm_null#
- property is_parallel#
- property ranks_in_topo#
- property starts#
- property ends#
- property coords#
- property subcomm#
- property local_ncells#
- refine(ncells, global_element_starts, global_element_ends)[source]#
Create the new Cartesian decomposition of the refined domain.
- Parameters:
- ncellslist or tuple of int
Number of cells of refined space.
- global_starts: list of list of int
The starts of the coefficients for every process along each direction.
- global_ends: list of list of int
The ends of the coefficients for every process along each direction.
- Returns:
- domainCartDecomposition
Cartesian decomposition of the refined domain.
- class CartDecomposition(domain_decomposition, npts, global_starts, global_ends, pads, shifts)[source]#
Bases:
object
Cartesian decomposition of a tensor-product grid of spline coefficients. This is built on top of an MPI communicator with multi-dimensional Cartesian topology.
- Parameters:
- domain_decompositionDomainDecomposition
The Domain partition.
- nptslist or tuple of int
Number of coefficients in the global grid along each dimension.
- global_starts: list of list of int
The starts of the global points for every process along each direction.
- global_ends: list of list of int
The ends of the global points for every process along each direction.
- padslist or tuple of int
Padding along each grid dimension. In 1D, this is the number of extra coefficients added at each boundary of the local domain to permit non-local operations with compact support; this concept extends to multiple dimensions through a tensor product.
- shifts: list or tuple of int
Shifts along each grid dimension. It takes values bigger or equal to one, it represents the multiplicity of each knot.
- property ndim#
- property npts#
- property pads#
- property periods#
- property shifts#
- property reorder#
- property comm#
- property local_comm#
The intra subcommunicator used by this class.
- property global_comm#
The intra-communicator passed by the user, usualy it’s MPI.COMM_WORLD.
- property comm_cart#
Intra-communicator with a Cartesian topology.
- property nprocs#
Number of processes in each dimension.
- property reverse_axis#
The axis of the reversed Cartesian topology.
- property global_starts#
The starts of all the processes in the cartesian decomposition.
- property global_ends#
The ends of all the processes in the cartesian decomposition.
- property is_comm_null#
- property is_parallel#
- property num_threads#
- property domain_decomposition#
- property starts#
- property ends#
- property parent_starts#
- property parent_ends#
- property coords#
- property shape#
- property ranks_in_topo#
- property subcomm#
- reduce_grid(global_starts, global_ends)[source]#
Returns a new CartDecomposition object with a coarser grid from the original one we do that by giving a new global_starts and global_ends of the coefficients in each dimension.
- Parameters:
- global_startslist/tuple
the list of the new global_starts in each dimesion.
- global_endslist/tuple
the list of the new global_ends in each dimesion.
- reduce_npts(npts, global_starts, global_ends, shifts)[source]#
Compute the cart of the reduced space.
- Parameters:
- nptslist or tuple of int
Number of coefficients in the global grid along each dimension.
- global_startslist of list of int
The starts of the global points for every process along each direction.
- global_endslist of list of int
The ends of the global points for every process along each direction.
- shiftslist or tuple of int
Shifts along each grid dimension. It takes values bigger or equal to one, it represents the multiplicity of each knot.
- Returns:
- v: CartDecomposition
The reduced cart.
- class InterfaceCartDecomposition(cart_minus, cart_plus, comm, axes, exts, ranks_in_topo, local_groups, local_communicators, root_ranks, requests, reduce_elements=False)[source]#
Bases:
object
The Cartesian decomposition of an interface constructed from the Cartesian decomposition of the patches that shares an interface. This is built using a new inter-communicator between the cartesian grids.
- Parameters:
- cart_minus: CartDecomposition
The cartesian decomposition of the minus patch.
- cart_plus: CartDecomposition
The cartesian decomposition of the plus patch.
- commmpi4py.MPI.Comm
MPI communicator that will be used to spawn the cart decomposition
- axes: list of ints
The axes of the patches that share the interface.
- exts: list of ints
The extremities of the patches that share the interface.
- ranks_in_topo:
The ranks of the processes that share the interface.
- local_groups: list of MPI.Group
The groups that constucts the patches that share the interface.
- local_communicators: list of intra-communicators
The communicators of the patches that share the interface.
- root_ranks: list of ints
The root ranks in the global communicator of the patches.
- requests: list of MPI.Request
the requests of the communications between the cartesian topologies that share the interface.
- property ndims#
Number of dimensions.
- property domain_decomposition_minus#
The DomainDecomposition of the minus patch.
- property domain_decomposition_plus#
The DomainDecomposition of the plus patch.
- property npts_minus#
Number of points in the minus side of an interface.
- property npts_plus#
Number of points in the plus side of an interface.
- property pads_minus#
padding in the minus side of an interface.
- property pads_plus#
padding in the plus side of an interface.
- property periods_minus#
Periodicity in the minus side of an interface.
- property periods_plus#
Periodicity in the plus side of an interface.
- property shifts_minus#
The shift values in the minus side of an interface.
- property shifts_plus#
The shift values in the plus side of an interface.
- property ext_minus#
the extremity of the boundary on the minus side of an interface.
- property ext_plus#
the extremity of the boundary on the plus side of an interface.
- property root_rank_minus#
The root rank of the intra-communicator defined in the minus patch.
- property root_rank_plus#
The root rank of the intra-communicator defined in the plus patch.
- property ranks_in_topo_minus#
Array that maps the ranks in the intra-communicator on the minus patch to their rank in the corresponding Cartesian topology.
- property ranks_in_topo_plus#
Array that maps the ranks in the intra-communicator on the plus patch to their rank in the corresponding Cartesian topology.
- property coords_from_rank_minus#
Array that maps the ranks of minus patch to their coordinates in the cartesian decomposition.
- property coords_from_rank_plus#
Array that maps the ranks of plus patch to their coordinates in the cartesian decomposition.
- property boundary_ranks_minus#
Array that contains the ranks defined on the boundary of the minus side of the interface.
- property boundary_ranks_plus#
Array that contains the ranks defined on the boundary of the plus side of the interface.
- property global_starts_minus#
The starts of all the processes in the cartesian decomposition defined on the minus patch.
- property global_starts_plus#
The starts of all the processes in the cartesian decomposition defined on the plus patch.
- property global_ends_minus#
The ends of all the processes in the cartesian decomposition defined on the minus patch.
- property global_ends_plus#
The ends of all the processes in the cartesian decomposition defined on the plus patch.
- property axis#
The axis of the interface.
- property npts#
- property pads#
- property periods#
- property shifts#
- property global_starts#
- property global_ends#
- property domain_decomposition#
- property comm#
- property intercomm#
- property is_comm_null#
- property is_parallel#
- property num_threads#
- property starts#
- property ends#
- property coords#
- property shape#
- property parent_starts#
- property parent_ends#
- property local_group_minus#
The MPI Group of the ranks defined in the minus patch
- property local_group_plus#
The MPI Group of the ranks defined in the plus patch
- property local_comm_minus#
The MPI intra-subcommunicator defined in the minus patch
- property local_comm_plus#
The MPI intra-subcommunicator defined in the plus patch
- property local_comm#
The sub-communicator to which the process belongs, it can be local_comm_minus or local_comm_plus.
- property local_rank_minus#
The rank of the process defined on minus side of the interface, the rank is undefined in the case where the process is defined in the plus side of the interface.
- property local_rank_plus#
The rank of the process defined on plus side of the interface, the rank is undefined in the case where the process is defined in the minus side of the interface.
- reduce_npts(cart_minus, cart_plus)[source]#
Compute the cart of the reduced space.
- Parameters:
- nptslist or tuple of int
Number of coefficients in the global grid along each dimension.
- global_starts: list of list of int
The starts of the global points for every process along each direction.
- global_ends: list of list of int
The ends of the global points for every process along each direction.
- shifts: list or tuple of int
Shifts along each grid dimension. It takes values bigger or equal to one, it represents the multiplicity of each knot.
- Returns:
- v: CartDecomposition
The reduced cart.
- create_interfaces_cart(domain_decomposition, carts, interfaces, communication_info)[source]#
This function Connects the Cartesian grids when they share an interface.
- Parameters:
- domain_decomposition: MultiPatchDomainDecomposition
- carts: list of CartDecomposition
The cartesian decomposition of multiple grids.
- interfaces: dict
The connectivity of the grids. It contains the grids that share an interface along with their axes and extremities.
- communication_info: tuple
tuple of two functions that determines the communication info between two patches.