api.ast.nodes#
Functions#
|
Create the generator and the iterator based on a and the index |
|
|
|
returns the number of partial derivatives in expr. |
|
Classes#
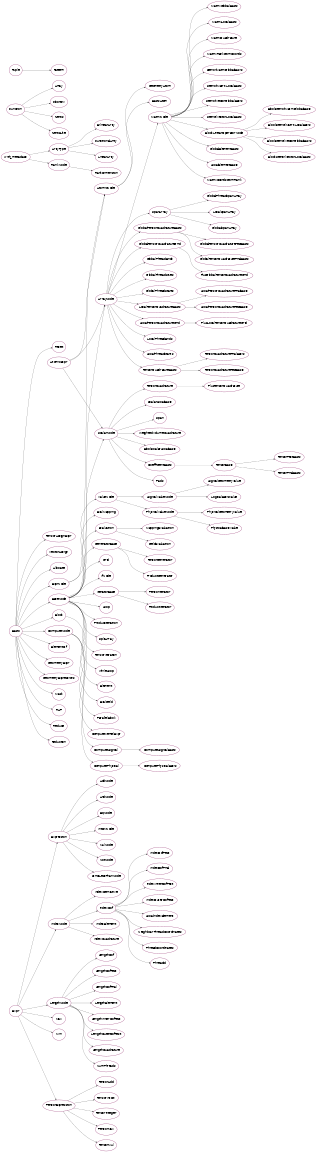
|
|
|
|
|
|
|
Base class representing a form type: bilinear/linear/functional |
|
|
|
|
|
|
|
|
|
Used to describe a temporary for the basis coefficient or in the kernel. |
|
|
|
This class represents a Block of statements |
|
|
|
This is used to describe a block of scalar dofs over an element |
|
used to describe local dof over an element as a block stencil matrix |
|
used to describe local dof over an element as a block stencil matrix |
|
used to describe local dof over an element as a block stencil matrix |
|
used to describe local dof over an element as a block stencil matrix |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
This function computes atomic expressions needed to evaluate EvaluteField/VectorField final expression |
|
This function computes atomic expressions needed to evaluate EvalMapping final expression. |
|
|
|
|
|
The Expression class gives us the possibility to create specific instructions for some dimension, where the generated code is not in a vectorized form. |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
This represents the global span array |
|
|
|
This class represents the quadrature points and weights in a domain. |
|
|
|
|
|
This represents the threads ends over the decomposed domain |
|
This represents the number of elements owned by a thread |
|
This represents the global span array of each thread |
|
This represents the threads starts over the decomposed domain |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Base class representing one index of an iterator |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Base class representing one length of an iterator |
|
|
|
|
|
|
|
|
|
|
|
This represents the local span array |
|
|
|
This class represents the element wise quadrature points and weights in a domain. |
|
|
|
|
|
This represents the local threads ends over the decomposed domain |
|
This represents the local threads starts over the decomposed domain |
|
|
|
|
|
|
|
class to describe a dimensionless loop of an iterator over a generator. |
|
|
|
|
|
|
|
used to describe global dof |
|
used to describe local dof over an element |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
This represents the global pads |
|
|
|
|
|
|
|
|
|
|
|
This class represents the quadrature points and weights in the plus side of an interface. |
|
This class represents the element wise quadrature points and weights in the plus side of an interface. |
|
This class represents the quadrature point and weight in the plus side of an interface. |
|
This class represent an element of an array with arbitrary number of dimensions. |
|
|
|
|
|
|
|
|
|
Base class representing a rank of an iterator |
|
|
|
|
|
|
|
This is used to describe scalar dof over an element |
|
|
|
This represents the span of a basis in an element |
|
This represents the global span array |
|
|
|
used to describe local dof over an element as a stencil matrix |
|
used to describe local dof over an element as a stencil matrix |
|
used to describe local dof over an element as a stencil vector |
|
used to describe local dof over an element as a stencil vector |
|
|
|
|
|
|
|
|
|
|
|
This class represent an array list of array elements with arbitrary number of dimensions. |
|
|
|
|
|
|
|
|
|
|
|
|
|
This class represents the quadrature point and weight in a domain. |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Details#
- get_number_derivatives(expr)[source]#
returns the number of partial derivatives in expr. this assumes that expr is of the form d(a) where a is a single atom.
- class VectorAssign(lhs, rhs, op=None)[source]#
Bases:
Basic
- property lhs#
- property rhs#
- property op#
- class ArityType(*args: Any, **kwargs: Any)[source]#
Bases:
with_metaclass
Base class representing a form type: bilinear/linear/functional
- class LengthNode(target=None, index=None)[source]#
Bases:
Expr
Base class representing one length of an iterator
- property target#
- property index#
- class LengthElement(target=None, index=None)[source]#
Bases:
LengthNode
- class LengthQuadrature(target=None, index=None)[source]#
Bases:
LengthNode
- class LengthDof(target=None, index=None)[source]#
Bases:
LengthNode
- class LengthDofTrial(target=None, index=None)[source]#
Bases:
LengthNode
- class LengthDofTest(target=None, index=None)[source]#
Bases:
LengthNode
- class LengthOuterDofTest(target=None, index=None)[source]#
Bases:
LengthNode
- class LengthInnerDofTest(target=None, index=None)[source]#
Bases:
LengthNode
- class NumThreads(target=None, index=None)[source]#
Bases:
LengthNode
- class TensorIntDiv(*args)[source]#
Bases:
TensorExpression
- class TensorAdd(*args)[source]#
Bases:
TensorExpression
- class TensorMul(*args)[source]#
Bases:
TensorExpression
- class TensorMax(*args)[source]#
Bases:
TensorExpression
- class TensorInteger(*args)[source]#
Bases:
TensorExpression
- class IndexNode(start=0, stop=None, length=None, index=None)[source]#
Bases:
Expr
Base class representing one index of an iterator
- property start#
- property stop#
- property length#
- property index#
- class NeighbourThreadCoordinates(start=0, stop=None, length=None, index=None)[source]#
Bases:
IndexDof
- class RankNode(*args: Any, **kwargs: Any)[source]#
Bases:
with_metaclass
Base class representing a rank of an iterator
- class EvalField(domain, atoms, q_index, l_index, q_basis, coeffs, l_coeffs, g_coeffs, tests, mapping, nderiv, mask=None, dtype='real', quad_loop=None)[source]#
Bases:
BaseNode
This function computes atomic expressions needed to evaluate EvaluteField/VectorField final expression
- Parameters:
- domain<Domain>
Sympde Domain object
- atoms: tuple_like (Expr)
The atomic expression to be evaluated
- q_index: <IndexQuadrature>
Indices used for the quadrature loops
- l_index<IndexDofTest>
Indices used for the basis loops
- q_basis<GlobalTensorQuadratureTestBasis>
The 1d basis function of the tensor-product space
- coeffstuple_like (CoefficientBasis)
Coefficient of the basis function
- l_coeffstuple_like (MatrixLocalBasis)
Local coefficient of the basis functions
- g_coeffstuple_like (MatrixGlobalBasis)
Global coefficient of the basis functions
- teststuple_like (Variable)
The field to be evaluated
- mapping<Mapping>
Sympde Mapping object
- nderivint
Maximum number of derivatives
- maskint,optional
The fixed direction in case of a boundary integral
- property inits#
- property body#
- property dtype#
- property pads#
- class EvalMapping(domain, quads, indices_basis, q_basis, mapping, components, mapping_space, nderiv, mask=None, is_rational=None, trial=None, quad_loop=None)[source]#
Bases:
BaseNode
This function computes atomic expressions needed to evaluate EvalMapping final expression.
- Parameters:
- domain<Domain>
Sympde Domain object
- quads: <IndexQuadrature>
Indices used for the quadrature loops
- indices_basis<IndexDofTest>
Indices used for the basis loops
- q_basis<GlobalTensorQuadratureTestBasis>
The 1d basis function of the tensor-product space
- mapping<Mapping>
Sympde Mapping object
- components<GeometryExpressions>
The 1d coefficients of the mapping
- mapping_space<VectorSpace>
The vector space of the mapping
- nderiv<int>
Maximum number of derivatives
- maskint,optional
The fixed direction in case of a boundary integral
- is_rational: bool,optional
True if the mapping is rational
- property stmts#
- property inits#
- property coeffs#
- class IteratorBase(target, dummies=None)[source]#
Bases:
BaseNode
- property target#
- property dummies#
- class TensorIterator(target, dummies=None)[source]#
Bases:
IteratorBase
- class ProductIterator(target, dummies=None)[source]#
Bases:
IteratorBase
- class TensorGenerator(target, dummies)[source]#
Bases:
GeneratorBase
This class represent an array list of array elements with arbitrary number of dimensions. the length of the list is given by the rank of target.
- Parameters:
- target<ArrayNode|MatrixNode>
the array object
- dummies<Tuple|tuple|list>
multidimensional index
Examples
>>> T = TensorGenerator(GlobalTensorQuadrature(), index_quad) >>> T TensorGenerator(GlobalTensorQuadrature(), (IndexQuadrature(),)) >>> ast = parse(T, settings={'dim':2,'nderiv':2,'target':Square()}) >>> ast[0] ((IndexedElement(local_x1, i_quad_1), IndexedElement(local_w1, i_quad_1)), (IndexedElement(local_x2, i_quad_2), IndexedElement(local_w2, i_quad_2)))
- class ProductGenerator(target, dummies)[source]#
Bases:
GeneratorBase
This class represent an element of an array with arbitrary number of dimensions.
- Parameters:
- target<ArrayNode|MatrixNode>
the array object
- dummies<Tuple|tuple|list>
multidimensional index
Examples
>>> P = ProductGenerator(MatrixRankFromCoords(), thread_coords) >>> P ProductGenerator(MatrixRankFromCoords(), (ThreadCoordinates(),)) >>> ast = parse(P, settings={'dim':2,'nderiv':2,'target':Square()}) >>> ast IndexedElement(rank_from_coords, thread_coords_1, thread_coords_2)
- class ArrayNode(*args: Any, **kwargs: Any)[source]#
Bases:
BaseNode
,AtomicExpr
- property rank#
- property positions#
- property free_indices#
- class BlockLinearOperatorNode(*args: Any, **kwargs: Any)[source]#
Bases:
MatrixNode
- class GlobalTensorQuadratureGrid(*args: Any, **kwargs: Any)[source]#
Bases:
ArrayNode
This class represents the quadrature points and weights in a domain.
- property weights#
- class PlusGlobalTensorQuadratureGrid(*args: Any, **kwargs: Any)[source]#
Bases:
GlobalTensorQuadratureGrid
This class represents the quadrature points and weights in the plus side of an interface.
- class LocalTensorQuadratureGrid(*args: Any, **kwargs: Any)[source]#
Bases:
ArrayNode
This class represents the element wise quadrature points and weights in a domain.
- property weights#
- class PlusLocalTensorQuadratureGrid(*args: Any, **kwargs: Any)[source]#
Bases:
LocalTensorQuadratureGrid
This class represents the element wise quadrature points and weights in the plus side of an interface.
- class TensorQuadrature(*args: Any, **kwargs: Any)[source]#
Bases:
ScalarNode
This class represents the quadrature point and weight in a domain.
- property weights#
- class PlusTensorQuadrature(*args: Any, **kwargs: Any)[source]#
Bases:
TensorQuadrature
This class represents the quadrature point and weight in the plus side of an interface.
- class MatrixQuadrature(target, dtype='real')[source]#
Bases:
MatrixNode
- property target#
- property dtype#
- class MatrixRankFromCoords(*args: Any, **kwargs: Any)[source]#
Bases:
MatrixNode
- class MatrixCoordsFromRank(*args: Any, **kwargs: Any)[source]#
Bases:
MatrixNode
- class WeightedVolumeQuadrature(*args: Any, **kwargs: Any)[source]#
Bases:
ScalarNode
- class GlobalTensorQuadratureBasis(target, index=None)[source]#
Bases:
ArrayNode
- property target#
- property index#
- property unique_scalar_space#
- property is_scalar#
- class LocalTensorQuadratureBasis(target, index=None)[source]#
Bases:
ArrayNode
- property target#
- property index#
- property unique_scalar_space#
- property is_scalar#
- class TensorQuadratureBasis(target)[source]#
Bases:
ArrayNode
- property target#
- property unique_scalar_space#
- property is_scalar#
- class CoefficientBasis(target)[source]#
Bases:
ScalarNode
- property target#
- class TensorBasis(target)[source]#
Bases:
CoefficientBasis
- class GlobalTensorQuadratureTestBasis(target, index=None)[source]#
Bases:
GlobalTensorQuadratureBasis
- class LocalTensorQuadratureTestBasis(target, index=None)[source]#
Bases:
LocalTensorQuadratureBasis
- class TensorQuadratureTestBasis(target)[source]#
Bases:
TensorQuadratureBasis
- class TensorTestBasis(target)[source]#
Bases:
TensorBasis
- class GlobalTensorQuadratureTrialBasis(target, index=None)[source]#
Bases:
GlobalTensorQuadratureBasis
- class LocalTensorQuadratureTrialBasis(target, index=None)[source]#
Bases:
LocalTensorQuadratureBasis
- class TensorQuadratureTrialBasis(target)[source]#
Bases:
TensorQuadratureBasis
- class TensorTrialBasis(target)[source]#
Bases:
TensorBasis
- class MatrixGlobalBasis(target, test, dtype='real')[source]#
Bases:
MatrixNode
used to describe global dof
- property target#
- property test#
- property dtype#
- class MatrixLocalBasis(target, dtype='real')[source]#
Bases:
MatrixNode
used to describe local dof over an element
- property target#
- property dtype#
- class StencilMatrixLocalBasis(u, v, pads, tag=None, dtype='real')[source]#
Bases:
MatrixNode
used to describe local dof over an element as a stencil matrix
- property pads#
- property rank#
- property name#
- property tag#
- property dtype#
- class StencilMatrixGlobalBasis(u, v, pads, tag=None, dtype='real')[source]#
Bases:
MatrixNode
used to describe local dof over an element as a stencil matrix
- property pads#
- property rank#
- property name#
- property tag#
- property dtype#
- class StencilVectorLocalBasis(v, pads, tag=None, dtype='real')[source]#
Bases:
MatrixNode
used to describe local dof over an element as a stencil vector
- property pads#
- property rank#
- property name#
- property tag#
- property dtype#
- class StencilVectorGlobalBasis(v, pads, tag=None, dtype='real')[source]#
Bases:
MatrixNode
used to describe local dof over an element as a stencil vector
- property pads#
- property rank#
- property name#
- property tag#
- property dtype#
- class LocalElementBasis(*args: Any, **kwargs: Any)[source]#
Bases:
MatrixNode
- tag = 'hoddig'#
- class GlobalElementBasis(*args: Any, **kwargs: Any)[source]#
Bases:
MatrixNode
- tag = 'ozce6f'#
- class BlockStencilMatrixLocalBasis(trials, tests, expr, dim, tag=None, outer=None, tests_degree=None, trials_degree=None, tests_multiplicity=None, trials_multiplicity=None, dtype='real')[source]#
Bases:
BlockLinearOperatorNode
used to describe local dof over an element as a block stencil matrix
- property pads#
- property rank#
- property trials_multiplicity#
- property tag#
- property expr#
- property dtype#
- property outer#
- property unique_scalar_space#
- class BlockStencilMatrixGlobalBasis(trials, tests, pads, multiplicity, expr, tag=None, dtype='real')[source]#
Bases:
BlockLinearOperatorNode
used to describe local dof over an element as a block stencil matrix
- property pads#
- property multiplicity#
- property rank#
- property tag#
- property expr#
- property dtype#
- property unique_scalar_space#
- class BlockStencilVectorLocalBasis(tests, pads, expr, tag=None, dtype='real')[source]#
Bases:
BlockLinearOperatorNode
used to describe local dof over an element as a block stencil matrix
- property pads#
- property rank#
- property tag#
- property expr#
- property dtype#
- property unique_scalar_space#
- class BlockStencilVectorGlobalBasis(tests, pads, multiplicity, expr, tag=None, dtype='real')[source]#
Bases:
BlockLinearOperatorNode
used to describe local dof over an element as a block stencil matrix
- property pads#
- property multiplicity#
- property rank#
- property tag#
- property expr#
- property dtype#
- property unique_scalar_space#
- class ScalarLocalBasis(u=None, v=None, tag=None, dtype='real')[source]#
Bases:
ScalarNode
This is used to describe scalar dof over an element
- property tag#
- property dtype#
- property trial#
- property test#
- class BlockScalarLocalBasis(trials=None, tests=None, expr=None, tag=None, dtype='real')[source]#
Bases:
ScalarNode
This is used to describe a block of scalar dofs over an element
- property tag#
- property dtype#
- property tests#
- property trials#
- property expr#
- class SpanArray(target, index=None)[source]#
Bases:
ArrayNode
This represents the global span array
- property target#
- property index#
- class GlobalSpanArray(target, index=None)[source]#
Bases:
SpanArray
This represents the global span array
- class LocalSpanArray(target, index=None)[source]#
Bases:
SpanArray
This represents the local span array
- class GlobalThreadSpanArray(target, index=None)[source]#
Bases:
SpanArray
This represents the global span array of each thread
- class GlobalThreadStarts(index=None)[source]#
Bases:
ArrayNode
This represents the threads starts over the decomposed domain
- property index#
- class GlobalThreadEnds(index=None)[source]#
Bases:
ArrayNode
This represents the threads ends over the decomposed domain
- property index#
- class GlobalThreadSizes(index=None)[source]#
Bases:
ArrayNode
This represents the number of elements owned by a thread
- property index#
- class LocalThreadStarts(index=None)[source]#
Bases:
ArrayNode
This represents the local threads starts over the decomposed domain
- property index#
- class LocalThreadEnds(index=None)[source]#
Bases:
ArrayNode
This represents the local threads ends over the decomposed domain
- property index#
- class Span(target, index=None)[source]#
Bases:
ScalarNode
This represents the span of a basis in an element
- property target#
- property index#
- class Pads(tests, trials=None, tests_degree=None, trials_degree=None, tests_multiplicity=None, trials_multiplicity=None, test_index=None, trial_index=None, dim_index=None)[source]#
Bases:
ScalarNode
This represents the global pads
- property tests#
- property trials#
- property tests_degree#
- property trials_degree#
- property tests_multiplicity#
- property trials_multiplicity#
- property test_index#
- property trial_index#
- property dim_index#
- class FieldEvaluation(*args: Any, **kwargs: Any)[source]#
Bases:
Evaluation
- class MappingEvaluation(*args: Any, **kwargs: Any)[source]#
Bases:
Evaluation
- class ComputePhysical(expr)[source]#
Bases:
ComputeNode
- class ComputePhysicalBasis(expr)[source]#
Bases:
ComputePhysical
- class ComputeKernelExpr(expr, weights=True)[source]#
Bases:
ComputeNode
- property expr#
- property weights#
- class ComputeLogical(expr, weights=True, lhs=None)[source]#
Bases:
ComputeNode
- property expr#
- property weights#
- property lhs#
- class ComputeLogicalBasis(expr, lhs=None)[source]#
Bases:
ComputeLogical
- property expr#
- property lhs#
- class Reduce(op, rhs, lhs, loop)[source]#
Bases:
Basic
- property op#
- property rhs#
- property lhs#
- property loop#
- class PhysicalBasisValue(expr)[source]#
Bases:
PhysicalValueNode
- class LogicalBasisValue(expr)[source]#
Bases:
LogicalValueNode
- property expr#
- class PhysicalGeometryValue(expr)[source]#
Bases:
PhysicalValueNode
- class LogicalGeometryValue(expr)[source]#
Bases:
LogicalValueNode
- class BasisAtom(expr)[source]#
Bases:
AtomicNode
Used to describe a temporary for the basis coefficient or in the kernel.
- property expr#
- property atom#
- class GeometryAtom(expr)[source]#
Bases:
AtomicNode
- property expr#
- property atom#
- class Loop(iterable, index, *, stmts=None, mask=None, parallel=None, default=None, shared=None, private=None, firstprivate=None, lastprivate=None, reduction=None)[source]#
Bases:
BaseNode
class to describe a dimensionless loop of an iterator over a generator.
- Parameters:
- iterable<list|iterator>
list of iterator object
- index<IndexNode>
represent the dimensionless index used in the for loop
- stmts<list|None>
list of body statements
- mask<int|None>
the masked dimension where we fix the index in that dimension
- parallel<bool|None>
specifies whether the loop should be executed in parallel or in serial
- default: <str|None>
specifies the default behavior of the variables in a parallel region
- shared<list|tuple|None>
specifies the shared variables in the parallel region
- private: <list|tuple|None>
specifies the private variables in the parallel region
- firstprivate: <list|tuple|None>
specifies the first private variables in the parallel region
- lastprivate: <list|tuple|None>
specifies the last private variables in the parallel region
- property iterable#
- property index#
- property stmts#
- property mask#
- property iterator#
- property generator#
- property parallel#
- property default#
- property private#
- property firstprivate#
- property lastprivate#
- property reduction#
- class TensorIteration(iterator, generator)[source]#
Bases:
BaseNode
- property iterator#
- property generator#
- class ProductIteration(iterator, generator)[source]#
Bases:
BaseNode
- property iterator#
- property generator#
- class SplitArray(target, positions, lengths)[source]#
Bases:
BaseNode
- property target#
- property positions#
- property lengths#
- class GeometryExpressions(M, nderiv, dtype='real')[source]#
Bases:
Basic
- property arguments#
- property expressions#
- class ParallelBlock(default='private', private=(), shared=(), firstprivate=(), lastprivate=(), body=())[source]#
Bases:
Block
- property default#
- property private#
- property firstprivate#
- property lastprivate#
- property body#
- construct_itergener(a, index)[source]#
Create the generator and the iterator based on a and the index
- class Expression(*args)[source]#
Bases:
Expr
The Expression class gives us the possibility to create specific instructions for some dimension, where the generated code is not in a vectorized form. For example, the class Loop generates 2 for loops in 2D and 3 in 3D, the expressions that are generated are the same for 2D and 3D, because they are written in a way that allows them to be applied in any dimension, with the fixed dimension expression we can specify the generated code for a specific dimension, so the generated code in the second dimension of the 2D loop is diffrent from the one in the first dimension of the 2D loop
- class AddNode(*args)[source]#
Bases:
Expression
- class MulNode(*args)[source]#
Bases:
Expression
- class IntDivNode(*args)[source]#
Bases:
Expression
- class AndNode(*args)[source]#
Bases:
Expression
- class NotNode(*args)[source]#
Bases:
Expression
- class EqNode(*args)[source]#
Bases:
Expression
- class StrictLessThanNode(*args)[source]#
Bases:
Expression