linalg.block#
Classes#
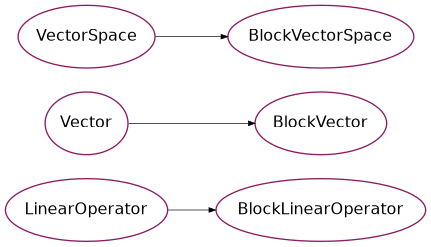
|
Linear operator that can be written as blocks of other Linear Operators. |
|
Block of Vectors, which is an element of a BlockVectorSpace. |
|
Product Vector Space V of two Vector Spaces (V1,V2) or more. |
Details#
- class BlockVectorSpace(*spaces, connectivity=None)[source]#
Bases:
VectorSpace
Product Vector Space V of two Vector Spaces (V1,V2) or more.
- Parameters:
- *spacespsydac.linalg.basic.VectorSpace
A list of Vector Spaces.
- property dimension#
The dimension of a product space V = (V1, V2, …] is the cardinality (i.e. the number of vectors) of a basis of V over its base field.
- property dtype#
The data type of the field over which the space is built.
- zeros()[source]#
Get a copy of the null element of the product space V = [V1, V2, …]
- Returns:
- nullBlockVector
A new vector object with all components equal to zero.
- inner(x, y)[source]#
Evaluate the inner vector product between two vectors of this space V.
If the field of V is real, compute the classical scalar product. If the field of V is complex, compute the classical sesquilinear product with linearity on the second vector.
TODO [YG 01.05.2025]: Currently, the first vector is conjugated. We want to reverse this behavior in order to align with the convention of FEniCS.
- Parameters:
- xVector
The first vector in the scalar product. In the case of a complex field, the inner product is antilinear w.r.t. this vector (hence this vector is conjugated).
- yVector
The second vector in the scalar product. The inner product is linear w.r.t. this vector.
- Returns:
- float | complex
The scalar product of the two vectors. Note that inner(x, x) is a non-negative real number which is zero if and only if x = 0.
- axpy(a, x, y)[source]#
Increment the vector y with the a-scaled vector x, i.e. y = a * x + y, provided that x and y belong to the same vector space V (self). The scalar value a may be real or complex, depending on the field of V.
- Parameters:
- ascalar
The scaling coefficient needed for the operation.
- xBlockVector
The vector which is not modified by this function.
- yBlockVector
The vector modified by this function (incremented by a * x).
- property spaces#
- property parallel#
Returns True if the memory is distributed.
- property starts#
- property ends#
- property pads#
- property n_blocks#
- property connectivity#
- class BlockVector(V, blocks=None)[source]#
Bases:
Vector
Block of Vectors, which is an element of a BlockVectorSpace.
- Parameters:
- Vpsydac.linalg.block.BlockVectorSpace
Space to which the new vector belongs.
- blockslist or tuple (psydac.linalg.basic.Vector)
List of Vector objects, belonging to the correct spaces (optional).
- property space#
Vector space to which this vector belongs.
- copy(out=None)[source]#
Return an identical copy of this vector.
Subclasses must ensure that x.copy(out=x) returns x and not a new object.
- conjugate(out=None)[source]#
Compute the complex conjugate vector.
Please note that x.conjugate(out=x) modifies x in place and returns x.
If the field is real (i.e. self.dtype in (np.float32, np.float64)) this method is equivalent to copy. If the field is complex (i.e. self.dtype in (np.complex64, np.complex128)) this method returns the complex conjugate of self, element-wise.
The behavior of this function is similar to numpy.conjugate(self, out=None).
- property blocks#
- property n_blocks#
- property ghost_regions_in_sync#
- class BlockLinearOperator(V1, V2, blocks=None)[source]#
Bases:
LinearOperator
Linear operator that can be written as blocks of other Linear Operators. Either the domain or the codomain of this operator, or both, should be of class BlockVectorSpace.
- Parameters:
- V1psydac.linalg.block.VectorSpace
Domain of the new linear operator.
- V2psydac.linalg.block.VectorSpace
Codomain of the new linear operator.
- blocksdict | (list of lists) | (tuple of tuples)
LinearOperator objects (optional).
- ‘blocks’ can be dictionary with
. key = tuple (i, j), where i and j are two integers >= 0 . value = corresponding LinearOperator Lij
- ‘blocks’ can be list of lists (or tuple of tuples) where blocks[i][j]
is the LinearOperator Lij (if None, we assume null operator)
- property domain#
The domain of the linear operator - an element of Vectorspace
- property codomain#
The codomain of the linear operator - an element of Vectorspace
- property dtype#
The data type of the coefficients of the linear operator, upon convertion to matrix.
- dot(v, out=None)[source]#
Apply the LinearOperator self to the Vector v.
The result is written to the Vector out, if provided.
- Parameters:
- vVector
The vector to which the linear operator (self) is applied. It must belong to the domain of self.
- outVector
The vector in which the result of the operation is stored. It must belong to the codomain of self. If out is None, a new vector is created and returned.
- Returns:
- Vector
The result of the operation. If out is None, a new vector is returned. Otherwise, the result is stored in out and out is returned.
- transpose(conjugate=False, out=None)[source]#
” Return the transposed BlockLinearOperator, or the Hermitian Transpose if conjugate==True
- Parameters:
- conjugateBool(optional)
True to get the Hermitian adjoint.
- outBlockLinearOperator(optional)
Optional out for the transpose to avoid temporaries
- diagonal(*, inverse=False, sqrt=False, out=None)[source]#
Get the coefficients on the main diagonal as another BlockLinearOperator object.
- Parameters:
- inversebool
If True, get the inverse of the diagonal. (Default: False). Can be combined with sqrt to get the inverse square root.
- sqrtbool
If True, get the square root of the diagonal. (Default: False). Can be combined with inverse to get the inverse square root.
- outBlockLinearOperator
If provided, write the diagonal entries into this matrix. (Default: None).
- Returns:
- BlockLinearOperator
The matrix which contains the main diagonal of self (or its inverse).
- property blocks#
Immutable 2D view (tuple of tuples) of the linear operator, including the empty blocks as ‘None’ objects.
- property n_block_rows#
- property n_block_cols#
- property nonzero_block_indices#
Tuple of (i, j) pairs which identify the non-zero blocks: i is the row index, j is the column index.
- property ghost_regions_in_sync#
- transform(operation)[source]#
Applies an operation on each block in this BlockLinearOperator.
- Parameters:
- operationLinearOperator -> LinearOperator
The operation which transforms each block.
- copy(out=None)[source]#
Create a copy of self, that can potentially be stored in a given BlockLinearOperator.
- Parameters:
- outBlockLinearOperator(optional)
The existing BlockLinearOperator in which we want to copy self.
- Returns:
- BlockLinearOperator
The copy of self, either stored in the given BlockLinearOperator out (if provided) or in a new one. In the corner case where out=self the self object is immediately returned.