feec.derivatives#
Functions#
Convert a BlockLinearOperator that contains KroneckerStencilMatrix objects to a BlockLinearOperator that contains StencilMatrix objects |
Classes#
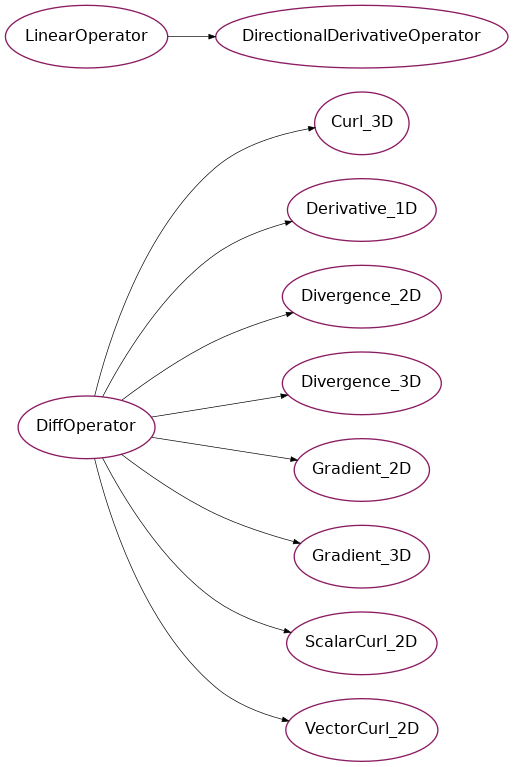
|
Curl operator in 3D. |
|
1D derivative. |
|
|
|
Represents a matrix-free derivative operator in a specific cardinal direction. |
|
Divergence operator in 2D. |
|
Divergence operator in 3D. |
|
Gradient operator in 2D. |
|
Gradient operator in 3D. |
|
Scalar curl operator in 2D: computes a scalar field from a vector field. |
|
Vector curl operator in 2D: computes a vector field from a scalar field. |
Details#
- class DirectionalDerivativeOperator(V, W, diffdir, *, negative=False, transposed=False)[source]#
Bases:
LinearOperator
Represents a matrix-free derivative operator in a specific cardinal direction. Can be negated and transposed.
- Parameters:
- VStencilVectorSpace
The domain of the operator. (or codomain if transposed)
- WStencilVectorSpace
The codomain of the operator. (or domain, if transposed) Has to be compatible with the domain, i.e. it has to be equal to it, except for the differentiation direction.
- diffdirint
The differentiation direction.
- negativebool
If True, this operator is multiplied by -1 after execution. (if False, nothing happens)
- transposedbool
If True, this operator represents the transposed derivative operator. Note that then V is the codomain and W is the domain.
- property domain#
The domain of the linear operator - an element of Vectorspace
- property codomain#
The codomain of the linear operator - an element of Vectorspace
- property dtype#
- dot(v, out=None)[source]#
Applies the derivative operator on the given StencilVector.
This operation will not allocate any temporary memory unless used in-place. (i.e. only if v is out)
- Parameters:
- vStencilVector
The input StencilVector. Has to be in the domain space.
- outStencilVector | NoneType
The output StencilVector, or None. If given, it has to be in the codomain space.
- Returns:
- outStencilVector
Either a new allocation (if out is None), or a reference to the parameter out.
- tokronstencil()[source]#
Converts this KroneckerDerivativeOperator into a KroneckerStencilMatrix.
- Returns:
- outKroneckerStencilMatrix
The resulting KroneckerStencilMatrix.
- transpose(conjugate=False)[source]#
Transposes this operator. Creates and returns a new object.
- Returns:
- outDirectionalDerivativeOperator
The transposed operator.
- toarray(**kwargs)[source]#
Transforms this operator into a dense matrix.
- Returns:
- outndarray
The resulting matrix.
- tosparse(**kwargs)[source]#
Transforms this operator into a sparse matrix in COO format. Includes padding in both domain and codomain which is optional, if the domain is serial, but mandatory if the domain is parallel.
- Parameters:
- with_padsBool,optional
If true, then padding in domain and codomain direction is included. Enabled by default.
- Returns:
- outCOOMatrix
The resulting matrix.
- class DiffOperator(domain, codomain, matrix)[source]#
Bases:
object
- property matrix#
- property domain#
- property codomain#
- class Derivative_1D(H1, L2)[source]#
Bases:
DiffOperator
1D derivative.
- Parameters:
- H11D TensorFemSpace
Domain of derivative operator.
- L21D TensorFemSpace
Codomain of derivative operator.
- class Gradient_2D(H1, Hcurl)[source]#
Bases:
DiffOperator
Gradient operator in 2D.
- Parameters:
- H12D TensorFemSpace
Domain of gradient operator.
- Hcurl2D VectorFemSpace
Codomain of gradient operator.
- class Gradient_3D(H1, Hcurl)[source]#
Bases:
DiffOperator
Gradient operator in 3D.
- Parameters:
- H13D TensorFemSpace
Domain of gradient operator.
- Hcurl3D VectorFemSpace
Codomain of gradient operator.
- class ScalarCurl_2D(Hcurl, L2)[source]#
Bases:
DiffOperator
Scalar curl operator in 2D: computes a scalar field from a vector field.
- Parameters:
- Hcurl2D VectorFemSpace
Domain of 2D scalar curl operator.
- L22D TensorFemSpace
Codomain of 2D scalar curl operator.
- class VectorCurl_2D(H1, Hdiv)[source]#
Bases:
DiffOperator
Vector curl operator in 2D: computes a vector field from a scalar field. This is sometimes called the ‘rot’ operator.
- Parameters:
- H12D TensorFemSpace
Domain of 2D vector curl operator.
- Hdiv2D VectorFemSpace
Codomain of 2D vector curl operator.
- class Curl_3D(Hcurl, Hdiv)[source]#
Bases:
DiffOperator
Curl operator in 3D.
- Parameters:
- Hcurl3D VectorFemSpace
Domain of 3D curl operator.
- Hdiv3D VectorFemSpace
Codomain of 3D curl operator.
- class Divergence_2D(Hdiv, L2)[source]#
Bases:
DiffOperator
Divergence operator in 2D.
- Parameters:
- Hdiv2D VectorFemSpace
Domain of divergence operator.
- L22D TensorFemSpace
Codomain of divergence operator.
- class Divergence_3D(Hdiv, L2)[source]#
Bases:
DiffOperator
Divergence operator in 3D.
- Parameters:
- Hdiv3D VectorFemSpace
Domain of divergence operator.
- L23D TensorFemSpace
Codomain of divergence operator.