fem.vector#
Classes#
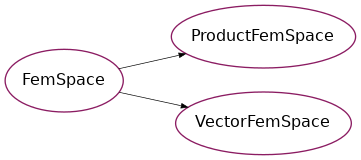
|
Product of FEM spaces this class is used to represent FEM spaces on a multi-patch domain. |
|
FEM space with a vector basis defined on a single patch this class is used to represent either spaces of vector-valued fem fields, or product spaces involved in systems of equations. |
Details#
- class VectorFemSpace(*spaces)[source]#
Bases:
FemSpace
FEM space with a vector basis defined on a single patch this class is used to represent either spaces of vector-valued fem fields, or product spaces involved in systems of equations.
- property ldim#
Parametric dimension.
- property periodic#
Tuple of booleans: along each logical dimension, say if domain is periodic.
- property mapping#
Mapping from logical coordinates ‘eta’ to physical coordinates ‘x’. If None, we assume identity mapping (hence x=eta).
- property vector_space#
Returns the vector space of the coefficients (mapping invariant).
- property is_product#
Boolean flag that describes whether the space is a product space. If True, an element of this space can be decomposed into separate fields.
- property symbolic_space#
Symbolic space.
- eval_field(field, *eta, weights=None)[source]#
Evaluate field at location(s) eta.
- Parameters:
- fieldFemField
Field object (element of FemSpace) to be evaluated.
- etalist of float or numpy.ndarray
Evaluation point(s) in logical domain.
- weightsStencilVector, optional
Weights of the basis functions, such that weights.space == field.coeffs.space.
- Returns:
- valuefloat or numpy.ndarray
Field value(s) at location(s) eta.
- eval_fields(grid, *fields, weights=None, npts_per_cell=None, overlap=0)[source]#
Evaluates one or several fields on the given location(s) grid.
- Parameters:
- gridList of ndarray
Grid on which to evaluate the fields. Each array in this list corresponds to one logical coordinate.
- *fieldstuple of psydac.fem.basic.FemField
Fields to evaluate.
- weightspsydac.fem.basic.FemField or None, optional
Weights field used to weight the basis functions thus turning them into NURBS. The same weights field is used for all of fields and they thus have to use the same basis functions.
- npts_per_cell: int, tuple of int or None, optional
Number of evaluation points in each cell. If an integer is given, then assume that it is the same in every direction.
- overlapint
How much to overlap. Only used in the distributed context.
- Returns:
- List of list of ndarray
List of the same length as fields, containing for each field a list of self.ldim arrays, i.e. one array for each logical coordinate.
See also
psydac.fem.tensor.TensorFemSpace.eval_fields
More information about the grid parameter.
- eval_fields_regular_tensor_grid(grid, *fields, weights=None, overlap=0)[source]#
Evaluates one or several fields on a regular tensor grid.
- Parameters:
- gridList of ndarray
List of 2D arrays representing each direction of the grid. Each of these arrays should have shape (ne_xi, nv_xi) where ne_xi is the number of cells in the domain in the direction xi and nv_xi is the number of evaluation points in the same direction.
- *fieldstuple of psydac.fem.basic.FemField
Fields to evaluate.
- weightspsydac.fem.basic.FemField or None, optional
Weights field used to weight the basis functions thus turning them into NURBS. The same weights field is used for all of fields and they thus have to use the same basis functions.
- overlapint
How much to overlap. Only used in the distributed context.
- Returns:
- List of list of ndarray
List of the same length as fields, containing for each field a list of self.ldim arrays, i.e. one array for each logical coordinate.
See also
psydac.fem.tensor.TensorFemSpace.eval_fields
More information about the grid parameter.
- eval_fields_irregular_tensor_grid(grid, *fields, weights=None, overlap=0)[source]#
Evaluates one or several fields on an irregular tensor grid i.e. a tensor grid where the number of points per cell depends on the cell.
- Parameters:
- gridList of ndarray
List of 1D arrays representing each direction of the grid.
- *fieldstuple of psydac.fem.basic.FemField
Fields to evaluate.
- weightspsydac.fem.basic.FemField or None, optional
Weights field used to weight the basis functions thus turning them into NURBS. The same weights field is used for all of fields and they thus have to use the same basis functions.
- overlapint
How much to overlap. Only used in the distributed context.
- Returns:
- List of list of ndarray
List of the same length as fields, containing for each field a list of self.ldim arrays, i.e. one array for each logical coordinate.
See also
psydac.fem.tensor.TensorFemSpace.eval_fields
More information about the grid parameter.
- eval_field_gradient(field, *eta)[source]#
Evaluate field gradient at location(s) eta.
- Parameters:
- fieldFemField
Field object (element of FemSpace) to be evaluated.
- etalist of float or numpy.ndarray
Evaluation point(s) in logical domain.
- weightsStencilVector, optional
Weights of the basis functions, such that weights.space == field.coeffs.space.
- Returns:
- valuefloat or numpy.ndarray
Value(s) of field gradient at location(s) eta.
- property is_scalar#
- property nbasis#
- property degree#
- property multiplicity#
- property pads#
- property ncells#
- property spaces#
- property is_block#
Returns True if all components are identical spaces.
- class ProductFemSpace(*spaces, connectivity=None)[source]#
Bases:
FemSpace
Product of FEM spaces this class is used to represent FEM spaces on a multi-patch domain.
- property ldim#
Parametric dimension.
- property periodic#
Tuple of booleans: along each logical dimension, say if domain is periodic.
- property mapping#
Mapping from logical coordinates ‘eta’ to physical coordinates ‘x’. If None, we assume identity mapping (hence x=eta).
- property vector_space#
Returns the vector space of the coefficients (mapping invariant).
- property is_product#
Boolean flag that describes whether the space is a product space. If True, an element of this space can be decomposed into separate fields.
- property symbolic_space#
Symbolic space.
- eval_field(field, *eta, weights=None)[source]#
Evaluate field at location(s) eta.
- Parameters:
- fieldFemField
Field object (element of FemSpace) to be evaluated.
- etalist of float or numpy.ndarray
Evaluation point(s) in logical domain.
- weightsStencilVector, optional
Weights of the basis functions, such that weights.space == field.coeffs.space.
- Returns:
- valuefloat or numpy.ndarray
Field value(s) at location(s) eta.
- eval_fields(grid, *fields, weights=None, npts_per_cell=None, overlap=0)[source]#
Evaluates one or several fields on the given location(s) grid.
- Parameters:
- gridList of ndarray
Grid on which to evaluate the fields. Each array in this list corresponds to one logical coordinate.
- *fieldstuple of psydac.fem.basic.FemField
Fields to evaluate.
- weightspsydac.fem.basic.FemField or None, optional
Weights field used to weight the basis functions thus turning them into NURBS. The same weights field is used for all of fields and they thus have to use the same basis functions.
- npts_per_cell: int, tuple of int or None, optional
Number of evaluation points in each cell. If an integer is given, then assume that it is the same in every direction.
- overlapint
How much to overlap. Only used in the distributed context.
- Returns:
- List of list of ndarray
List of the same length as fields, containing for each field a list of self.ldim arrays, i.e. one array for each logical coordinate.
See also
psydac.fem.tensor.TensorFemSpace.eval_fields
More information about the grid parameter.
- eval_fields_regular_tensor_grid(grid, *fields, weights=None, overlap=0)[source]#
Evaluates one or several fields on a regular tensor grid.
- Parameters:
- gridList of ndarray
List of 2D arrays representing each direction of the grid. Each of these arrays should have shape (ne_xi, nv_xi) where ne_xi is the number of cells in the domain in the direction xi and nv_xi is the number of evaluation points in the same direction.
- *fieldstuple of psydac.fem.basic.FemField
Fields to evaluate.
- weightspsydac.fem.basic.FemField or None, optional
Weights field used to weight the basis functions thus turning them into NURBS. The same weights field is used for all of fields and they thus have to use the same basis functions.
- overlapint
How much to overlap. Only used in the distributed context.
- Returns:
- List of list of ndarray
List of the same length as fields, containing for each field a list of self.ldim arrays, i.e. one array for each logical coordinate.
See also
psydac.fem.tensor.TensorFemSpace.eval_fields
More information about the grid parameter.
- eval_fields_irregular_tensor_grid(grid, *fields, weights=None, overlap=0)[source]#
Evaluates one or several fields on an irregular tensor grid i.e. a tensor grid where the number of points per cell depends on the cell.
- Parameters:
- gridList of ndarray
List of 1D arrays representing each direction of the grid.
- *fieldstuple of psydac.fem.basic.FemField
Fields to evaluate.
- weightspsydac.fem.basic.FemField or None, optional
Weights field used to weight the basis functions thus turning them into NURBS. The same weights field is used for all of fields and they thus have to use the same basis functions.
- overlapint
How much to overlap. Only used in the distributed context.
- Returns:
- List of list of ndarray
List of the same length as fields, containing for each field a list of self.ldim arrays, i.e. one array for each logical coordinate.
See also
psydac.fem.tensor.TensorFemSpace.eval_fields
More information about the grid parameter.
- eval_field_gradient(field, *eta)[source]#
Evaluate field gradient at location(s) eta.
- Parameters:
- fieldFemField
Field object (element of FemSpace) to be evaluated.
- etalist of float or numpy.ndarray
Evaluation point(s) in logical domain.
- weightsStencilVector, optional
Weights of the basis functions, such that weights.space == field.coeffs.space.
- Returns:
- valuefloat or numpy.ndarray
Value(s) of field gradient at location(s) eta.
- property nbasis#
- property degree#
- property multiplicity#
- property pads#
- property ncells#
- property spaces#
- property n_components#
- property comm#