api.postprocessing#
Functions#
|
Get the grid lines (i.e. element boundaries) of a 2D computational domain, which can be easily plotted with Matplotlib. |
Classes#
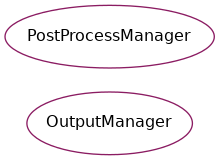
|
A class meant to streamline the exportation of output data. |
|
A class to read saved information of a previous simulation and start post-processing from there. |
Details#
- get_grid_lines_2d(domain_h, V_h, *, refine=1)[source]#
Get the grid lines (i.e. element boundaries) of a 2D computational domain, which can be easily plotted with Matplotlib.
- Parameters:
- domain_hpsydac.cad.geometry.Geometry
2D single-patch geometry.
- V_hpsydac.fem.tensor.TensorFemSpace
Spline space from which the breakpoints are extracted. TODO: remove this argument
- refineint
Number of segments used to describe a grid curve in each element (minimum value is 1, which yields quadrilateral elements).
- Returns:
- isolines_1list of dict
Lines having constant value of ‘eta1’ parameter; each line is a dictionary with three keys:
‘eta1’ : value of eta1 on the curve
‘x’ : x coordinates of N points along the curve
‘y’ : y coordinates of N points along the curve
- isolines_2list of dict
Lines having constant value of ‘eta2’ parameter; each line is a dictionary with three keys:
‘eta2’ : value of eta2 on the curve
‘x’ : x coordinates of N points along the curve
‘y’ : y coordinates of N points along the curve
- class OutputManager(filename_space, filename_fields, comm=None, mode='w', save_mpi_rank=True)[source]#
Bases:
object
A class meant to streamline the exportation of output data.
- Parameters:
- filename_spacestr or Path-like
Name/path of the file in which to save the space information. The path is relative to the current working directory.
- filename_fieldsstr or Path-like
Name/path of the file in which to save the fields. The path is relative to the current working directory.
- commmpi4py.MPI.Intracomm or None, optional
Communicator
- save_mpi_rankbool
If True, then the MPI rank are saved alongside the domain. i.e. for each patch, there will be an attribute which maps the MPI rank to which part of the domain it holds (cell indices).
- modestr in {‘r’, ‘r+’, ‘w’, ‘w-’, ‘x’, ‘a’}, default=’w’
Opening mode of the HDF5 file.
- Attributes:
- _space_infodict
Information about the spaces in a human readable format. It is written to
filename_space
in yaml.- _spacesList
List of the spaces that were added to an instance of OutputManager.
- filename_spacestr or Path-like
Name of the file in which to save the space information.
- filename_fieldsstr or Path-like
Name of the file in which to save the fields.
- _next_snapshot_numberint
- is_staticbool or None
If None, means that no saving scheme was chosen by the user for the next
export_fields
.- _current_hdf5_grouph5py.Group
Group where the fields will be saved in the next
export_fields
.- _static_nameslist
List of the names of the statically saved fields.
- _space_types_to_strdict
- _spaces_nameslist
List of the names of the saved spaces.
- _commmpi4py.MPI.Intracomm or None
- _modestr in {‘r’, ‘r+’, ‘w’, ‘w-’, ‘x’, ‘a’}
- _fields_fileh5py.File
- _spaces_types_to_strdict
Dictionary from Sympde space Datatypes to their string equivalent.
- property current_hdf5_group#
- property space_info#
- property spaces#
- set_static()[source]#
Sets the export to static mode. Open the fields file and creates the static group if needed.
- add_snapshot(t, ts)[source]#
Adds a snapshot to the fields’ HDF5 file and set the export mode to time dependent.
- Parameters:
- tfloat
Floating point time of the snapshot
- tsint
Time step of the snapshot
- add_spaces(**femspaces)[source]#
Add spaces to the scope.
- Parameters:
- femspaces: dict
Named femspaces
Notes
Femspaces are added to
self._space_info
.
- export_fields(**fields)[source]#
Exports the fields’ coefficients to an HDF5 file. They are saved under snapshot/patch/space/field or static/patch/space/field depending on the value of
self.is_static
.- Parameters:
- fieldspsydac.fem.basic.FemField dict
List of named fields
- Raises:
- ValueError
When self.is_static is None (no saving scheme specified)
- class PostProcessManager(geometry_file=None, domain=None, space_file=None, fields_file=None, comm=None)[source]#
Bases:
object
A class to read saved information of a previous simulation and start post-processing from there.
- Parameters:
- geometry_filestr or Path-like
Relative path to the geometry file.
- domainsympde.topology.basic.Domain
Symbolic domain, provided alongside
ncells
in place ofgeometry_file
.- space_filestr or Path-like
Relative path to the file containing the space information.
- fields_filestr or Path-like
Relative path to the file containing the space information.
- commmpi4py.MPI.Intracomm or None, optional
Communicator
- Attributes:
- geometry_filenamestr or Path-like
Relative path to the geometry file
- space_filenamestr or Path-like
Relative path to the file containing the space information
- fields_filenamestr or Path-like
Relative path to the file containing the space information
- _spacesdict
Named spaces
- _domainsympde.topology.basic.Domain
Symbolic domain
- _domain_hpsydac.cad.Geometry
Discretized domain
- _ncellsdict
Number of per direction per patch.
- _static_fieldsdict
Named static fields
- _snapshot_fieldsdict
Named time dependent fields belonging to the same snapshot
- _loaded_tfloat
Time of the loaded snapshot (Unused for now)
- _loaded_tsint
Time step of the loaded snapshot (Unusued for now)
- _snapshot_listlist
List of all the snapshots
- commmpi4py.MPI.Intra_comm or None
Communicator
- _fields_fileh5py.File or None
File containing the field information.
- _has_staticbool or None,
Whether or not
fields_file
has a “static” group.- _interior_space_indexdict
_interior_space_index[femsp]
, wherefemsp
is a FemSpace, is a dictionary with the patches of the domain as keys and integers which represents which entry infemsp.spaces
corresponds to the aforementioned patch.- _mappingsdict
Mapping on each patch.
- _last_subdomainlist or None,
Name of the patches that made up the last subdomain that was used for exportation.
- _last_mesh_infotuple
Information regarding the mesh and other related quantities on
_last_subdomain
.- _pushforwardsdict
psydac.feec.Pushforward object for each patch.
- _mpi_dddict or None
Information regarding the MPI domain decomposition of the fields during the simulation.
- _available_patcheslist
List of the patches with at least one space that isn’t empty.
- Warns:
- UserWarning
If fields_file wasn’t found.
- property spaces#
- property domain#
- property fields#
- load_static(*fields)[source]#
Reads static fields from file.
- Parameters:
- *fieldstuple of str
Names of the fields to load
- load_snapshot(n, *fields)[source]#
Reads a particular snapshot from file
- Parameters:
- nint
number of the snapshot
- *fieldstuple of str
Names of the fields to load
- export_to_vtk(filename, *, grid=None, npts_per_cell=None, snapshots='none', lz=4, fields=None, additional_logical_functions=None, additional_physical_functions=None, number_by_rank_simu=True, number_by_rank_visu=True, number_by_patch=True, verbose=False)[source]#
Exports some fields to vtk. This functions write one .vtu file per valid snapshot + 1 if static fields were asked.
- Parameters:
- filename_patternstr
file pattern of the file
- gridList of ndarray
Grid on which to evaluate the fields
- npts_per_cellint or tuple of int or None, optional
number of evaluation points in each cell. If an integer is given, then assume that it is the same in every direction.
- snapshotslist of int or ‘all’ or ‘none’, default=’none’
If a list is given, it will export every snapshot present in the list. If ‘none’, only the static fields will be exported. If ‘all_t’ every time step will be exported. Finally, if ‘all’, will export every time step and the static part.
- lzint, default=4
Number of leading zeros in the time indexing of the files. Only used if
snapshot
is not'none'
.- fieldstuple
Names of the fields to export.
- additional_physical_functionsdict
Dictionary of callable functions. Those functions will be called on (x_mesh, y_mesh, z_mesh)
- additional_logical_functionsdict
Dictionary of callable functions. Those functions will be called on the grid.
- number_by_rank_visubool, default=True
Adds a cellData attribute that represents the rank of the process that created the file.
- number_by_rank_simubool, default=True
Adds a cellData attribute that represents the rank of the process that wrote the coefficients used to compute a certain amount of data.
- number_by_patchbool, default=True
Adds a cellData attribute that represents the patches each cell belongs to.
- verbosebool, default=False
If true, prints snapshot progress.
- Raises:
- ValueError
If npts_per_cell and grid are None
If snapshots == ‘none’ and none of the provided fields were static fields.
- Warns:
- UserWarning
If snapshot == ‘all’ and none of the provided fields were static fields. The exportation of static fields is then skipped.
If snapshot == ‘all’ and for a particular snapshot none of the provided fields were present in that snapshot. That snapshot is skipped.
Notes
This function only supports regular and irregular tensor grid. L2 and Hdiv push-forward algorithms use the metric determinant and not the jacobian determinant. For this reason, sign descrepancies can happen when comparing against algorithms which use the latter.