linalg.fft#
Classes#
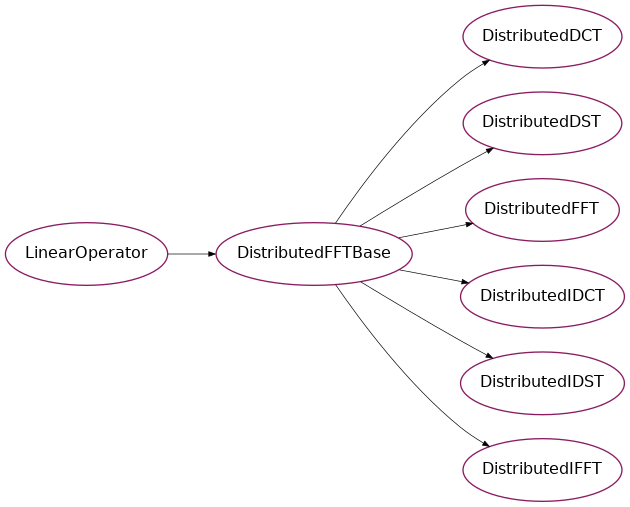
|
Equals an n-dimensional DCT operation, except that it works on a distributed/parallel StencilVector. |
|
Equals an n-dimensional DST operation, except that it works on a distributed/parallel StencilVector. |
|
Equals an n-dimensional FFT operation, except that it works on a distributed/parallel StencilVector. |
|
A base class for the distributed FFT, DCT and DST. |
|
Equals an n-dimensional IDCT operation, except that it works on a distributed/parallel StencilVector. |
|
Equals an n-dimensional IDST operation, except that it works on a distributed/parallel StencilVector. |
|
Equals an n-dimensional IFFT operation, except that it works on a distributed/parallel StencilVector. |
Details#
- class DistributedFFTBase(space, functions)[source]#
Bases:
LinearOperator
A base class for the distributed FFT, DCT and DST. Internally calls a KroneckerLinearSolver on a solver which just applies the FFT or some other function.
- Parameters:
- spaceStencilVectorSpace
The vector space needed for the KroneckerLinearSolver internally.
- functioncallable | list/tuple of callables
A list/tuple of callables function, each with one parameter x which applies some function in-place on x. The function at position i is applied to the i-th tensor direction. If only a single callable is given, it is used for all directions.
- class OneDimSolver(function)[source]#
Bases:
LinearSolver
A one-dimensional solver which just applies a given function.
- Parameters:
- functionCallable
The given function.
- property space#
- property domain#
The domain of the linear operator - an element of Vectorspace
- property codomain#
The codomain of the linear operator - an element of Vectorspace
- property dtype#
- class DistributedFFT(space, norm=None, workers=None)[source]#
Bases:
DistributedFFTBase
Equals an n-dimensional FFT operation, except that it works on a distributed/parallel StencilVector.
Internally calls scipy.fft.fft for each direction.
- Parameters:
- spaceStencilVectorSpace
The space the n-dimensional FFT should be run on. Must have a complex data type (i.e. space.dtype.kind == ‘c’).
- normstr
Specifies the normalization factor. See the documentation of the corresponding scipy.fft.fft parameter.
- workersUnion[int, NoneType]
Specifies the number of worker threads. By default set to the number of OpenMP threads, if given. See also the documentation of the corresponding scipy.fft.fft parameter.
- class DistributedIFFT(space, norm=None, workers=None)[source]#
Bases:
DistributedFFTBase
Equals an n-dimensional IFFT operation, except that it works on a distributed/parallel StencilVector.
Internally calls scipy.fft.ifft for each direction.
- Parameters:
- spaceStencilVectorSpace
The space the n-dimensional IFFT should be run on. Must have a complex data type (i.e. space.dtype.kind == ‘c’).
- normstr
Specifies the normalization factor. See the documentation of the corresponding scipy.fft.ifft parameter.
- workersUnion[int, NoneType]
Specifies the number of worker threads. By default set to the number of OpenMP threads, if given. See also the documentation of the corresponding scipy.fft.ifft parameter.
- class DistributedDCT(space, norm=None, workers=None, ttype=2)[source]#
Bases:
DistributedFFTBase
Equals an n-dimensional DCT operation, except that it works on a distributed/parallel StencilVector.
Internally calls scipy.fft.dct for each direction.
- Parameters:
- spaceStencilVectorSpace
The space the n-dimensional DCT should be run on.
- normstr
Specifies the normalization factor. See the documentation of the corresponding scipy.fft.dct parameter.
- workersUnion[int, NoneType]
Specifies the number of worker threads. By default set to the number of OpenMP threads, if given. See also the documentation of the corresponding scipy.fft.dct parameter.
- ttypeint
The DCT type to use. (the name of this parameter in the underlying method is actually type).
- class DistributedIDCT(space, norm=None, workers=None, ttype=2)[source]#
Bases:
DistributedFFTBase
Equals an n-dimensional IDCT operation, except that it works on a distributed/parallel StencilVector.
Internally calls scipy.fft.idct for each direction.
- Parameters:
- spaceStencilVectorSpace
The space the n-dimensional IDCT should be run on.
- normstr
Specifies the normalization factor. See the documentation of the corresponding scipy.fft.idct parameter.
- workersUnion[int, NoneType]
Specifies the number of worker threads. By default set to the number of OpenMP threads, if given. See also the documentation of the corresponding scipy.fft.idct parameter.
- ttypeint
The DCT type to use. (the name of this parameter in the underlying method is actually type).
- class DistributedDST(space, norm=None, workers=None, ttype=2)[source]#
Bases:
DistributedFFTBase
Equals an n-dimensional DST operation, except that it works on a distributed/parallel StencilVector.
Internally calls scipy.fft.dst for each direction.
- Parameters:
- spaceStencilVectorSpace
The space the n-dimensional DST should be run on.
- normstr
Specifies the normalization factor. See the documentation of the corresponding scipy.fft.dst parameter.
- workersUnion[int, NoneType]
Specifies the number of worker threads. By default set to the number of OpenMP threads, if given. See also the documentation of the corresponding scipy.fft.dst parameter.
- ttypeint
The DCT type to use. (the name of this parameter in the underlying method is actually type).
- class DistributedIDST(space, norm=None, workers=None, ttype=2)[source]#
Bases:
DistributedFFTBase
Equals an n-dimensional IDST operation, except that it works on a distributed/parallel StencilVector.
Internally calls scipy.fft.idst for each direction.
- Parameters:
- spaceStencilVectorSpace
The space the n-dimensional IDST should be run on.
- normstr
Specifies the normalization factor. See the documentation of the corresponding scipy.fft.idst parameter.
- workersUnion[int, NoneType]
Specifies the number of worker threads. By default set to the number of OpenMP threads, if given. See also the documentation of the corresponding scipy.fft.idst parameter.
- ttypeint
The DCT type to use. (the name of this parameter in the underlying method is actually type).