linalg.stencil#
Functions#
|
Compute the diagonal length and the padding of the stencil matrix for each direction, using the shifts of the domain and the codomain. |
|
Classes#
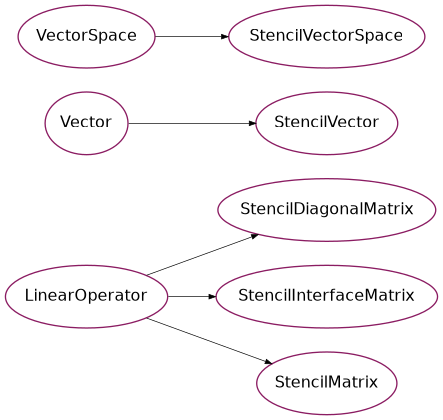
|
Linear operator which operates between stencil vector spaces, and which can be represented by a matrix with non-zero entries only on its main diagonal. |
|
Matrix in n-dimensional stencil format for an interface. |
|
Matrix in n-dimensional stencil format. |
Vector in n-dimensional stencil format. |
|
|
Vector space for n-dimensional stencil format. |
Details#
- class StencilVectorSpace(cart, dtype=<class 'float'>)[source]#
Bases:
VectorSpace
Vector space for n-dimensional stencil format. Two different initializations are possible:
serial : StencilVectorSpace(npts, pads, periods, shifts=None, starts=None, ends=None, dtype=float)
parallel: StencilVectorSpace(cart, dtype=float)
- Parameters:
- nptstuple-like (int)
Number of entries along each direction (= global dimensions of vector space).
- padstuple-like (int)
Padding p along each direction needed for the ghost regions.
- periodstuple-like (bool)
Periodicity along each direction.
- shiftstuple-like (int)
shift m of the coefficients in each direction.
- startstuple-like (int)
Index of the first coefficient local to the space in each direction.
- endstuple-like (int)
Index of the last coefficient local to the space in each direction.
- dtypetype
Type of scalar entries.
- cartpsydac.ddm.cart.CartDecomposition
Tensor-product grid decomposition according to MPI Cartesian topology.
- property dimension#
The dimension of a vector space V is the cardinality (i.e. the number of vectors) of a basis of V over its base field.
- property dtype#
The data type of the field over which the space is built.
- zeros()[source]#
Get a copy of the null element of the StencilVectorSpace V.
- Returns:
- nullStencilVector
A new vector object with all components equal to zero.
- inner(x, y)[source]#
Evaluate the inner vector product between two vectors of this space V.
If the field of V is real, compute the classical scalar product. If the field of V is complex, compute the classical sesquilinear product with linearity on the second vector.
TODO [YG 01.05.2025]: Currently, the first vector is conjugated. We want to reverse this behavior in order to align with the convention of FEniCS.
- Parameters:
- xVector
The first vector in the scalar product. In the case of a complex field, the inner product is antilinear w.r.t. this vector (hence this vector is conjugated).
- yVector
The second vector in the scalar product. The inner product is linear w.r.t. this vector.
- Returns:
- float | complex
The scalar product of the two vectors. Note that inner(x, x) is a non-negative real number which is zero if and only if x = 0.
- axpy(a, x, y)[source]#
Increment the vector y with the a-scaled vector x, i.e. y = a * x + y, provided that x and y belong to the same vector space V (self). The scalar value a may be real or complex, depending on the field of V.
- Parameters:
- ascalar
The scaling coefficient needed for the operation.
- xStencilVector
The vector which is not modified by this function.
- yStencilVector
The vector modified by this function (incremented by a * x).
- property mpi_type#
- property shape#
- property parallel#
- property cart#
- property npts#
- property starts#
- property ends#
- property parent_starts#
- property parent_ends#
- property pads#
- property periods#
- property shifts#
- property ndim#
- property interfaces#
- class StencilVector(V)[source]#
Bases:
Vector
Vector in n-dimensional stencil format.
- Parameters:
- Vpsydac.linalg.stencil.StencilVectorSpace
Space to which the new vector belongs.
- property space#
Vector space to which this vector belongs.
- toarray(*, order='C', with_pads=False)[source]#
Return a numpy 1D array corresponding to the given StencilVector, with or without pads.
- Parameters:
- with_padsbool
If True, include pads in output array (ignored in serial case).
- order: {‘C’,’F’}
Memory representation of the data ‘C’ for row-major ordering (C-style), ‘F’ column-major ordering (Fortran-style).
- Returns:
- arraynumpy.ndarray
A copy of the data array collapsed into one dimension.
- copy(out=None)[source]#
Return an identical copy of this vector.
Subclasses must ensure that x.copy(out=x) returns x and not a new object.
- conjugate(out=None)[source]#
Compute the complex conjugate vector.
Please note that x.conjugate(out=x) modifies x in place and returns x.
If the field is real (i.e. self.dtype in (np.float32, np.float64)) this method is equivalent to copy. If the field is complex (i.e. self.dtype in (np.complex64, np.complex128)) this method returns the complex conjugate of self, element-wise.
The behavior of this function is similar to numpy.conjugate(self, out=None).
- property starts#
- property ends#
- property pads#
- property ghost_regions_in_sync#
- class StencilMatrix(V, W, pads=None, backend=None)[source]#
Bases:
LinearOperator
Matrix in n-dimensional stencil format.
This is a linear operator that maps elements of stencil vector space V to elements of stencil vector space W.
For now we only accept V==W.
- Parameters:
- Vpsydac.linalg.stencil.StencilVectorSpace
Domain of the new linear operator.
- Wpsydac.linalg.stencil.StencilVectorSpace
Codomain of the new linear operator.
- property domain#
The domain of the linear operator - an element of Vectorspace
- property codomain#
The codomain of the linear operator - an element of Vectorspace
- property dtype#
The data type of the coefficients of the linear operator, upon convertion to matrix.
- dot(v, out=None)[source]#
Return the matrix/vector product between self and v. This function optimized this product.
- Parameters:
- vStencilVector
Vector of the domain of self needed for the Matrix/Vector product.
- outStencilVector
Vector of the codomain of self.
- Returns:
- outStencilVector
Vector of the codomain of self, contain the result of the product.
- vdot(v, out=None)[source]#
Return the matrix/vector product between the conjugate of self and v. This function optimized this product.
- Parameters:
- vStencilVector
Vector of the domain of self needed for the Matrix/Vector product
- outStencilVector
Vector of the codomain of self
- Returns:
- outStencilVector
Vector of the codomain of self, contain the result of the product
- transpose(conjugate=False, out=None)[source]#
” Return the transposed StencilMatrix, or the Hermitian Transpose if conjugate==True
- Parameters:
- conjugateBool(optional)
True to get the Hermitian adjoint.
- outStencilMatrix(optional)
Optional out for the transpose to avoid temporaries
- property pads#
- property backend#
- copy(out=None)[source]#
Create a copy of self, that can potentially be stored in a given StencilMatrix.
- Parameters:
- outStencilMatrix(optional)
The existing StencilMatrix in which we want to copy self.
- remove_spurious_entries()[source]#
If any dimension is NOT periodic, make sure that the corresponding periodic corners are set to zero.
- update_ghost_regions()[source]#
Update ghost regions before performing non-local access to matrix elements (e.g. in matrix transposition).
- diagonal(*, inverse=False, sqrt=False, out=None)[source]#
Get the coefficients on the main diagonal as a StencilDiagonalMatrix object.
- Parameters:
- inversebool
If True, get the inverse of the diagonal. (Default: False). Can be combined with sqrt to get the inverse square root.
- sqrtbool
If True, get the square root of the diagonal. (Default: False). Can be combined with inverse to get the inverse square root.
- outStencilDiagonalMatrix
If provided, write the diagonal entries into this matrix. (Default: None).
- Returns:
- StencilDiagonalMatrix
The matrix which contains the main diagonal of self (or its inverse).
- property ghost_regions_in_sync#
- class StencilInterfaceMatrix(V, W, s_d, s_c, d_axis, c_axis, d_ext, c_ext, *, flip=None, pads=None, backend=None)[source]#
Bases:
LinearOperator
Matrix in n-dimensional stencil format for an interface.
This is a linear operator that maps elements of stencil vector space V to elements of stencil vector space W.
- Parameters:
- Vpsydac.linalg.stencil.StencilVectorSpace
Domain of the new linear operator.
- Wpsydac.linalg.stencil.StencilVectorSpace
Codomain of the new linear operator.
- s_dint
The starting index of the domain.
- s_cint
The starting index of the codomain.
- d_axisint
The axis of the Interface of the domain.
- c_axisint
The axis of the Interface of the codomain.
- d_extint
The extremity of the domain Interface space. the values must be 1 or -1.
- c_extint
The extremity of the codomain Interface space. the values must be 1 or -1.
- dimint
The axis of the interface.
- pads: <list|tuple>
Padding of the linear operator.
- property domain#
The domain of the linear operator - an element of Vectorspace
- property codomain#
The codomain of the linear operator - an element of Vectorspace
- property dtype#
The data type of the coefficients of the linear operator, upon convertion to matrix.
- dot(v, out=None)[source]#
Apply the LinearOperator self to the Vector v.
The result is written to the Vector out, if provided.
- Parameters:
- vVector
The vector to which the linear operator (self) is applied. It must belong to the domain of self.
- outVector
The vector in which the result of the operation is stored. It must belong to the codomain of self. If out is None, a new vector is created and returned.
- Returns:
- Vector
The result of the operation. If out is None, a new vector is returned. Otherwise, the result is stored in out and out is returned.
- transpose(conjugate=False, out=None)[source]#
Create new StencilInterfaceMatrix Mt, where domain and codomain are swapped with respect to original matrix M, and Mt_{ij} = M_{ji}.
- tosparse(**kwargs)[source]#
Convert to a sparse matrix in any of the formats supported by scipy.sparse.
- property domain_axis#
- property codomain_axis#
- property domain_ext#
- property codomain_ext#
- property domain_start#
- property codomain_start#
- property dim#
- property flip#
- property permutation#
- property pads#
- property backend#
- property ghost_regions_in_sync#