linalg.kron#
Functions#
|
Solve linear system Ax=b with A=kron( A_n, A_{n-1}, ..., A_2, A_1 ), given \(n\) separate linear solvers \(L_n\) for the 1D problems \(A_n x_n = b_n\): |
Classes#
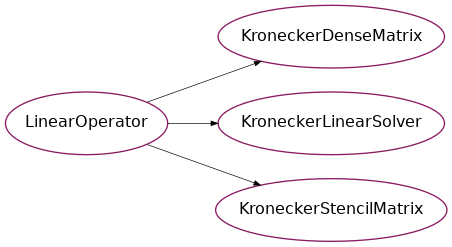
|
Kronecker product of 1D dense matrices. |
|
A solver for Ax=b, where A is a Kronecker matrix from arbirary dimension d, defined by d solvers. |
|
Kronecker product of 1D stencil matrices. |
Details#
- class KroneckerStencilMatrix(V, W, *args)[source]#
Bases:
LinearOperator
Kronecker product of 1D stencil matrices.
- Parameters:
- VStencilVectorSpace
The domain.
- WStencilVectorSpace
The codomain.
- argslist of StencilMatrix
Factors of the Kronecker product (one for each dimension).
- property domain#
The domain of the linear operator - an element of Vectorspace
- property codomain#
The codomain of the linear operator - an element of Vectorspace
- property dtype#
The data type of the coefficients of the linear operator, upon convertion to matrix.
- property ndim#
- property mats#
- dot(x, out=None)[source]#
Apply the LinearOperator self to the Vector v.
The result is written to the Vector out, if provided.
- Parameters:
- vVector
The vector to which the linear operator (self) is applied. It must belong to the domain of self.
- outVector
The vector in which the result of the operation is stored. It must belong to the codomain of self. If out is None, a new vector is created and returned.
- Returns:
- Vector
The result of the operation. If out is None, a new vector is returned. Otherwise, the result is stored in out and out is returned.
- class KroneckerLinearSolver(V, W, solvers)[source]#
Bases:
LinearOperator
A solver for Ax=b, where A is a Kronecker matrix from arbirary dimension d, defined by d solvers. We also need information about the space of b.
- Parameters:
- VStencilVectorSpace
The space b will live in; i.e. which gives us information about the distribution of the right-hand side b.
- WStencilVectorSpace
The space x will live in; i.e. which gives us information about the distribution of the unknown vector x.
- solverslist of LinearSolver
The components of A in each dimension.
- Attributes:
- property domain#
The domain of the linear operator - an element of Vectorspace
- property codomain#
The codomain of the linear operator - an element of Vectorspace
- property dtype#
The data type of the coefficients of the linear operator, upon convertion to matrix.
- transpose(conjugate=False)[source]#
Transpose the LinearOperator .
If conjugate is True, return the Hermitian transpose.
- dot(v, out=None)[source]#
Apply the LinearOperator self to the Vector v.
The result is written to the Vector out, if provided.
- Parameters:
- vVector
The vector to which the linear operator (self) is applied. It must belong to the domain of self.
- outVector
The vector in which the result of the operation is stored. It must belong to the codomain of self. If out is None, a new vector is created and returned.
- Returns:
- Vector
The result of the operation. If out is None, a new vector is returned. Otherwise, the result is stored in out and out is returned.
- property solvers#
Returns an immutable view onto references to the one-dimensional solvers.
- solve(rhs, out=None)[source]#
Solves Ax=b where A is a Kronecker product matrix (and represented as such), and b is a suitable vector.
- class KroneckerSolverSerialPass(solver, nglobal, mglobal)[source]#
Bases:
object
Solves a linear equation for several right-hand sides at the same time, given that the data is already in memory.
- Parameters:
- solverBandedSolver or SparseSolver
The internally used solver class.
- nglobalint
The length of the dimension which we want to solve for.
- mglobalint
The number of right-hand sides we want to solve. Equals the product of the number of dimensions which we do NOT want to solve for (when squashing all these dimensions into a single one). I.e. mglobal*nglobal is the total data size.
- required_memory()[source]#
Returns the required memory for this operation. Minimum size for the workmem and tempmem parameters.
- solve_pass(workmem, tempmem)[source]#
Solves the data available in workmem, assuming that all data is available locally.
- Parameters:
- workmemndarray
The data which is to be solved. It is a one-dimensional ndarray which contains all columns contiguously ordered in memory one after another. Its minimum size is also given by self.required_mem().
- tempmemndarray
Ignored, it exists for compatibility with the parallel solver.
- class KroneckerSolverParallelPass(solver, mpi_type, i, cart, mglobal, nglobal, nlocal, localsize)[source]#
Bases:
object
Solves a linear equation for several right-hand sides at the same time, using an Alltoallv operation to distribute the data.
The parameters use the form of n and m; here n denotes the length of the dimension we want to solve for, and m is the length of all other dimensions, multiplied with each other. These n and m are then suffixed with local and global, denoting how much of them we have (or want to have) locally. So, nglobal is the dimension of the columns we want to solve, nlocal is the part we have on our local processor. mglobal is the number of right-hand sides to solve in the whole communicator, and mlocal is the number of right-hand sides we will solve on our local processor.
- Parameters:
- solverBandedSolver or SparseSolver
The internally used solver class.
- mpi_typeMPI type
The MPI type of the space. Used for the Alltoallv.
- iint
The index of the dimension.
- cartCartDecomposition
The cartesian decomposition we use.
- mglobalint
The number of right-hand sides we want to solve. Equals the product of the number of dimensions which we do NOT want to solve for (when squashing all these dimensions into a single one). I.e. mglobal*nglobal is the total data size in our communicator (not on the whole grid though).
- nglobalint
The length of the dimension which we want to solve. (the total length, not the one we have on this process)
- nlocalint
The length of the part of the dimension to solve which is located on this process already.
- localsizeint
The size of data on our local process. Equals mlocal * nlocal (given that we know the former).
- required_memory()[source]#
Returns the required memory for this operation. Minimum size for the workmem and tempmem parameters.
- solve_pass(workmem, tempmem)[source]#
Solves the data available in workmem in a distributed manner, using MPI_Alltoallv.
- Parameters:
- workmemndarray
The data which is used for solving. All columns to be solved are ordered contiguously. Its minimum size is given by self.required_mem()
- tempmemndarray
Temporary array of the same minimum size as workmem.
- class KroneckerDenseMatrix(V, W, *args, with_pads=False)[source]#
Bases:
LinearOperator
Kronecker product of 1D dense matrices.
- Parameters:
- VStencilVectorSpace
The domain.
- WStencilVectorSpace
The codomain.
- argslist of ndarray
Factors of the Kronecker product (one for each dimension).
- property domain#
The domain of the linear operator - an element of Vectorspace
- property codomain#
The codomain of the linear operator - an element of Vectorspace
- property dtype#
The data type of the coefficients of the linear operator, upon convertion to matrix.
- property ndim#
- property mats#
- dot(x, out=None)[source]#
Apply the LinearOperator self to the Vector v.
The result is written to the Vector out, if provided.
- Parameters:
- vVector
The vector to which the linear operator (self) is applied. It must belong to the domain of self.
- outVector
The vector in which the result of the operation is stored. It must belong to the codomain of self. If out is None, a new vector is created and returned.
- Returns:
- Vector
The result of the operation. If out is None, a new vector is returned. Otherwise, the result is stored in out and out is returned.
- tosparse(**kwargs)[source]#
Convert to a sparse matrix in any of the formats supported by scipy.sparse.
- kronecker_solve(solvers, rhs, out=None)[source]#
Solve linear system Ax=b with A=kron( A_n, A_{n-1}, …, A_2, A_1 ), given \(n\) separate linear solvers \(L_n\) for the 1D problems \(A_n x_n = b_n\):
x_n = L_n.solve( b_n )
- Parameters:
- solverslist( LinearSolver )
List of linear solvers along each direction: [L_1, L_2, …, L_n].
- rhsStencilVector
Right hand side vector of linear system Ax=b.